Tutorial on how to use modules in Python like import, create, document and define classes with scripts examples.
This part of the Python course is about the Python modules. A module in Python is simply a file that contains definitions, functions, and statements. Here’s a detailed explanation of how modules work in Python, with code examples to illustrate each concept:
Table of Contents
1. Import and use built-in modules in Python
Python comes with a variety of built-in modules that provide a wide range of functionality. These modules are imported using the “import” statement. For example, to import the built-in “math” module, you would write “import math” at the top of your script.
import math
Once the module is imported, you can use its functions by calling them with the module’s name as a prefix. For example, to use the “sqrt” function from the math module, you would call “math.sqrt(4)”.
This allows you to perform mathematical operations such as square root, trigonometric functions and many more. By importing a module, you can use its functions and constants, and make use of the functionalities it provides without having to rewrite them.
# Import the built-in math module import math # Use the sqrt function from the math module print(math.sqrt(9)) # output : 3.0 # Importing sin function from math module from math import sin print(sin(90)) #output : 0.8939... # Using alias while importing a module import math as m print(m.sqrt(16)) # output : 4.0
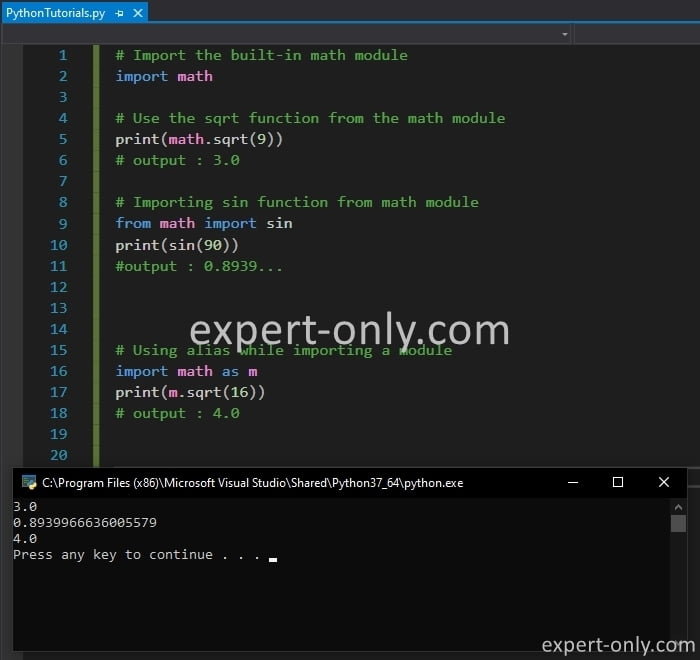
In the first example, we use the import statement to import the built-in “math” module. Then we can use the sqrt function from the math module by calling math.sqrt(9). The output will be 3.0..
2. Import specific functions from a Python module
You can also import specific functions or variables from a module using the from
keyword. For example, to import only the sqrt
function from the math module, you would write from math import sqrt
. This allows you to call the function directly without having to use the module’s name as a prefix. This means you can simply write sqrt(4)
instead of math.sqrt(4)
. By doing this way, you can import and use only the functions and variable you need, and avoid unnecessary imports.
# Import only the sqrt function from the math module from math import sqrt # Use the sqrt function print(sqrt(4)) # output: 2.0 # Importing multiple functions from the math module from math import sin, cos print(sin(60)) # output : 0.8660254037844386 print(cos(45)) # output : 0.5253219888177297 # Importing variables from math import pi print(pi) # output : 3.141592653589793
The goal when import only specific functions or Python variables, is to save memory and make code more easy to read.
In the first example, we use the from
keyword to import only the sqrt
function from the math module. This allows us to call the function directly without having to use the module’s name as a prefix. so we can simply write sqrt(4)
instead of math.sqrt(4)
In the second example, we import multiple functions from the math module using a single line. This allows you to call the functions directly without having to use the module’s name as a prefix. so we can simply write sin(60)
and cos(45)
instead of math.sin(60)
and math.cos(45)
.
In the third example, we imported the variable pi
from math module, which can be used directly without any prefix.
This other tutorial from the Python full course is about the user-defined functions in Python.
3. Assign aliases to modules or functions in Python
Python allows you to assign an alias to a module or function when you import it. This can be useful when you have multiple modules or functions with similar or duplicate names, or when you want to shorten the name of a module or function for easier reference.
This is done using the as
keyword. For example, you could import the math
module and assign it the alias m
like this: import math as m
. Then you can use the module’s functions by calling them with the alias as a prefix, like this m.sqrt(4)
instead of math.sqrt(4)
.
# Import the math module and give it an alias import math as m # Use the sqrt function from the math module with the alias print(m.sqrt(4)) # output: 2.0 # Use alias while importing a function from math import sin as sine print(sine(90)) # output : 1.0
In the first example, we import the math
module and give it the alias m
, so we can use the math
module functions by calling them with the alias as a prefix, like this m.sqrt(4)
instead of math.sqrt(4)
. In the second example, we use the as
keyword to import only the sin
function from the math module and giving it an alias sine
.
This allows you to call the function directly with the alias as a prefix, like this sine(90)
instead of math.sin(90)
This way you can use the more meaningful and descriptive names for the functions and variables in your code, while keeping the original names of the imported modules and functions.
4. Access functions and variables in Python modules
In Python, modules can contain variables and functions that can be accessed by other modules. These are called global variables and functions. To access these variables and functions, you can use the module name as a prefix, followed by a dot and the name of the variable or function.
For example, consider a module named mymodule
that contains the following variable:
x = 3
You could access the value of x
from another module as follows:
import mymodule print(mymodule.x) # Output: 3
Similarly, consider a module named mymodule
that contains the following function:
def print_hello(): print("Hello, World!")
You could call the print_hello
function from another module as follows:
import mymodule mymodule.print_hello() # Output: "Hello, World!"
It’s important to note that global variables and functions are not visible to the modules that import them by default, it has to be explicitly imported using the import statement.
5. Create and use custom Python modules
In addition to the built-in modules that come with Python, you can also create your own modules by writing your own Python scripts and saving them with the .py
file extension. To use your own module in another script, you’ll need to put the module file in the same directory as the script that imports it, or in a directory listed in the sys.path
list.
Here is an example of a custom module mymodule.py
:
def print_hello(): print("Hello, World!") def print_goodbye(): print("Goodbye, World!")
And an example of how to use it in another Python script, this one prints two message in the output screen:
- Hello, World!
- Goodbye, World!
import mymodule mymodule.print_hello() # Output: "Hello, World!" mymodule.print_goodbye() # Output: "Goodbye, World!"
In case your custom module is in different directory from the script, you will have to use sys.path
to add the directory to the path list.
import sys sys.path.append("/path/to/your/module") import mymodule mymodule.print_hello() # Output: "Hello, World!" mymodule.print_goodbye() # Output: "Goodbye, World!"
It’s important to note that naming convention in python is to use all lowercase letters, and to use _ as word delimiter, it’s a good practice to follow it to prevent any naming conflicts when using multiple modules.
This other tutorial presents the multiple data types available with Python programming language.
6. How to create and use packages in Python?
A Python package is a way to organize related modules together. To create a package, follow these steps:
- First create a directory.
- Include an __init__.py file within the directory.
The presence of the __init__.py
file indicates that the directory should be treated as a package by Python. Here is an example package directory structure that contains 2 Python modules.
myfirstpackage/ __init__.py module1.py module2.py
The __init__.py
file can be used to initialize the package or to define some variables or functions which will be shared across all modules of the package.
# __init__.py shared_variable = "This is a shared variable" def print_hello(): print("Hello from mypackage")
When package is imported, __init__.py
is executed, in this way you can share variables and functions across all modules of package.
import mypackage print(mypackage.shared_variable) # Output: "This is a shared variable" mypackage.print_hello() # Output: "Hello from mypackage"
It is also possible to import a specific module and use variables and functions defined in it, like in the code below.
from mypackage import module1 module1.print_hello() # Output: "Hello from module1"
It’s important to note that the init.py file can be empty as well, it serves only as a marker for python to recognize the directory as a package.
7. Document Python modules with docstrings
A module in Python can have a docstring, which is a string that describes what the module does. Docstrings can be a useful tool for documentation and can be accessed by other developers to understand the purpose and functionality of a module.
The docstring is placed as the first statement in a module and is accessible using the __doc__
attribute. Here’s an example of a module with a docstring:
""" This module contains functions for performing mathematical operations. """ def add(a, b): """ Add two numbers """ return a + b def multiply(a, b): """ Multiply two numbers """ return a * b
You can access the docstring using the __doc__
attribute, for example, in the script below, we display the documentation of the math_operations module.
import math_operations print(math_operations.__doc__) # Output: # This module contains functions for performing mathematical operations.
You can also access the docstring of a given function, to do so, add the function name inside the print function, like this.
print(math_operations.add.__doc__) # Output: # Add two numbers
It’s good practice in Python programming to use docstring and provide clear and comprehensive documentation in your modules. This will make it easier for other developers to understand and use your code.
8. Execute statements in Python modules
Python modules can also include executable statements, which are executed when the module is first imported. These statements are typically used to define global variables and functions, or to perform other one-time setup tasks. The following script example use a module with executable statements.
x = 3 def print_hello(): print("Hello, World!") print("Module is being imported")
When this module is imported for the first time, the statements x = 3
, def print_hello():
and print("Module is being imported")
will be executed. The variable x
and the function print_hello
will be available for use, and the string “Module is being imported” will be printed.
import mymodule print(mymodule.x) # Output: 3 mymodule.print_hello() # Output: "Hello, World!"
It’s important to note that these statements are executed only once, when the module is imported for the first time, not every time when it’s imported later. It’s also good practice to keep these statements separate from the function and class definitions to make the code more readable and maintainable.
9. Define classes in Python modules
Python modules can also include classes, which can be used to create objects of a particular type. For example, you might create a class in a module that represents a particular type of data, such as a Customer record or a Person record. This module uses a script that defines a class called Customer
. The Customer class below can manage these 7 fields:
- First name
- Last name
- Address
- Phone
- Profession
- Purchases (in the form of a Python list)
class Customer: def __init__(self, first_name, last_name, address, phone, email, profession): self.first_name = first_name self.last_name = last_name self.address = address self.phone = phone self.email = email self.profession = profession self.purchases = [] def display_customer_info(self): """ Display Customer Information """ print(f"Name: {self.first_name} {self.last_name}") print(f"Profession: {self.profession}") print(f"Address: {self.address}") print(f"Phone: {self.phone}") print(f"Email: {self.email}") if self.purchases: print("Purchases:") for purchase in self.purchases: print(purchase) else: print("No purchases made yet") def update_address(self, new_address): """ update the customer address """ self.address = new_address def make_purchase(self, item): """ make purchase and add to customer's purchase history """ self.purchases.append(item)
Once a class has been defined in a module, you can create objects of that class using the class’s constructor and use the methods associated with the Customer class.
import mymodule cust = mymodule.Customer("John", "Smith", "1st Ave, NY", "555-555-5555", "johnsmith@example.com") cust.display_customer_info() # Output: # Name: John Smith # Address: 1st Ave, NY # Phone: 555-555-5555 # Email: johnsmith@example.com cust.update_address("2nd Ave, NY") cust.display_customer_info() # Output: # Name: John Smith # Address: 2nd Ave, NY # Phone: 555-555-5555 # Email: johnsmith@example.com
It’s a good practice in Python and in OO Programming to keep the classes and modules related to each other. And also use the class name as the module name, that way it’s easy to understand what classes a module contains and also easy to locate the module while importing.
10. Use the main block in modules
Python modules can include a special block of code, called the if __name__ == "__main__"
block. This block of code will only be executed if the module is run as the main script. This can be useful for testing purposes, or for providing a way to run the module as a standalone script.
def my_function(): print("This is my function") if __name__ == "__main__": my_function()
The if __name__ == "__main__"
block is an incredibly useful tool for any Python developer. It allows you to easily check whether your module is being run as a standalone script or being imported as a module in another script. If the module is being run as a standalone script, the function inside the block will be called and executed.
But if the same module is imported in another script, the block will not be executed, allowing you to separate your code for different purposes. This means you can use the if __name__ == "__main__"
block to test your modules separately, without having to run your whole project.
It’s also great for creating standalone scripts that can run independently of other scripts in your project. It’s an elegant solution that ensures that your code runs exactly as it should, depending on the context it’s being used in. It’s a simple but powerful technique that can really improve the organization and maintainability of your code.
Conclusion on working with Python modules
That is for this interesting tutorial on how to work with Python modules. We have seen some of the powerful possibilities with examples of scripts to create and use modules.
In conclusion, Python modules are a powerful tool for organizing and structuring your code. They allow you to separate different functionality into different files, making your code more manageable and easier to understand. We’ve covered the basics of importing and using modules, as well as some of the advanced features such as importing specific Python functions, giving modules aliases, accessing global variables and functions, and using condition on the block named main.
The ability to import and use pre-built modules can save a lot of time and effort, as it allows you to take advantage of the work that others have already done. It also makes it easy to share and reuse your own code, by packaging it into a module that others can import and use in their own projects.
Furthermore, understanding the concept of classes and objects, and how to create and use them in Python modules, can help you create more powerful and organized programs.
All in all, Python modules are a fundamental part of the language, and mastering their use is an important step in becoming a proficient Python developer. With the knowledge you have gained from this tutorial, you can now start building more complex and powerful Python programs with confidence and enthusiasm. Good luck!
Popular MS tutorials from the blog (Power BI, T-SQL and MS-DOS)
- Calculate the last year value in Power BI with DAX
- Calculate the difference between two dates with SQL Server
- Insert or remove SQL Server line breaks from text
- Rename multiple Windows files at the same time
Be the first to comment