Tutorial to learn how to create user-defined functions in Python using arguments, scope and recursivity.
Functions, including user-defined functions in Python, are blocks of organized, reusable code that are given a name and used to perform a specific task. They provide modularity and code reuse in programming. They are used to perform a specific task, and they can be called upon whenever that task is needed. Functions are a fundamental building block of Python and are an essential part of writing clean, efficient, and reusable code.
This tutorial is following the two previous ones, about the introduction to Python programming, followed by the Python data types, and the last one about how to use the Python basic syntax to write code.
In this course, you will learn about different types of functions in Python, including built-in functions and user-defined functions. But also anonymous functions, also called lambda functions, and recursive ones. You will also learn about function arguments, including required arguments, keyword arguments, default arguments, and variable-length arguments.
You will also learn about the scope of variables, including global variables and local variables. Finally, you will learn about modules and packages, which are used to organize and reuse code in Python.
Table of Contents
1. Introduction to user-defined Python functions
User-defined functions are inspired by mathematical functions, where it is the definition of steps that gives a specific result from an argument.
A function is a block of organized, reusable code that is used to perform a single, related action. Functions provide better modularity for your application and a high degree of code reusing. As you already know, Python gives you many built-in functions like print(), etc. but you can also create your own functions. These functions are called user-defined functions.
2. Define a function in Python
You can create user-defined Python functions to provide a required, specific functionality not provided by the built-in functions. Here are simple rules to define a function in Python programming.
- Function blocks begin with the keyword def followed by the function name and parentheses ( ( ) ).
- Any input parameters or arguments should be placed within these parentheses. You can also define parameters inside these parentheses.
- The first statement of a function can be an optional statement – the documentation string of the function or docstring.
- The code block within every function starts with a colon (:) and is indented.
- The statement return [expression] exits a function, optionally passing back an expression to the caller. A return statement with no arguments is the same as return None.
Here is an example of a user-defined function that takes two numbers as arguments and returns their sum.
def sum(a, b): # This function returns the sum of two numbers result = a + b return result
Calling a Function Defining a function only gives it a name, specifies the parameters that are to be included in the function’s definition, and structures the blocks of code.
To execute a function, you must call it from somewhere else in your code. You call a function by using the function name followed by parentheses. Consider this first example.
result = sum(1, 2) print(result) # output is : 3
3. User-defined Python functions arguments
You can call a user-defined function by using the following 4 types of formal arguments:
- Required arguments
- Keyword arguments
- Default arguments
- Variable-length arguments
3.1 Required arguments
Required arguments are the arguments passed to a function in correct positional order. Here, the number of arguments in the function call should match exactly with the function definition.
def greet(name): # This function greets to the person passed in as parameter # by printing Hello followed by the person's name print("Hello, " + name + ". Good morning!") greet('Paul') # The output is Hello, Paul. Good morning!
3.2 Keyword arguments
Keyword arguments are related to the function calls. When you use keyword arguments in a function call, the caller identifies the arguments by the parameter name.
def greet(name, greeting): """This function greets to the person with the provided greeting""" print(greeting + ", " + name) greet(greeting = "Hello", name = "Paul") # output will be : Hello, Paul
3.3 Default arguments
A default argument is an argument that assumes a default value if a value is not provided in the function call for that argument. The following example gives an idea on default arguments, it prints default age if it is not passed.
def welcome(name, age = 28): # This function write welcome to the person with the provided name. # and prints its age print("Welcome, " + name + ". You are " + str(age) + " years old.") welcome("Ahmed") # output of the code : Hello, Ahmed. You are 28 years old.
You can also specify the value of an argument in the function call. In this case, the default value is overridden.
welcome("Ahmed", 32) # In this case, the output will be: Welcome, Ahmed. You are 32 years old.
3.4 Variable-length arguments
You may need to process a function for more arguments than you specified while defining the function. These arguments are called variable-length arguments and are not named in the function definition, unlike required and default arguments.
An asterisk (*) is placed before the variable name that holds the values of all non-keyword variable arguments. This tuple remains empty if no additional arguments are specified during the function call.
def greet(*names): # This function greets all the persons in the names tuple # names is a tuple with arguments for name in names: print("Welcome, " + name) greet("Ahmed", "Lee", "Monica", "Luke", "Steve", "John") # the output of the line of code above is Welcome, Ahmed Welcome, Lee Welcome, Monica Welcome, Luke Welcome, Steve Welcome, John
4. Scope of variables in Python
All variables in a program may not be accessible at all locations in that program. This depends on where you have declared a variable. The scope of a variable determines the portion of the program where you can access a particular identifier.
There are two basic scopes of variables in Python:
- Local variables
- Global variables
4.1 Local Variables
A local variable is a variable that is defined inside a function. It can only be accessed from within the function in which it is defined. Here is an example of how to create and use a local variable in Python:
def foo(): x = "local" print("x inside :", x) foo() print("x outside:", x) # Output x inside : local Traceback (most recent call last): File "<stdin>", line 7, in <module> NameError: name 'x' is not defined
4.2 Global Variables
A global variable is a variable that is defined outside of a function. It can be accessed from anywhere in the code, both inside and outside of functions. Check this other practical code example to create and use a Python global variable.
x = "global" def foo(): print("x inside :", x) foo() print("x outside:", x) # Output x inside : global x outside: global
5. Anonymous Functions (Lambda Functions)
Sometimes, you need to write a small function for a short period of time. In these cases, you can use an anonymous function, also known as a lambda function. Lambda functions are small functions without a name. They are defined using the lambda keyword, which has the following syntax:
Here is an example of a lambda function in Python:
double = lambda x: x * 2 print(double(5)) # Output is 10
In this example, the lambda function double
takes in one argument x
and returns x * 2
. When we call the function with the argument 5
, it returns 10
. Here is another example of a lambda function that takes in two arguments and returns the sum.
sum = lambda x, y: x + y print(sum(3, 4)) # the output is 7
It is also possible to use lambda functions as arguments of other Python functions, built-in or user-defined. For example, the following uses the sort
function to sort a list of tuples based on the second element of each tuple.
my_list = [(1, 2), (3, 1), (5, 4)] my_list.sort(key = lambda x: x[1]) print(my_list)
This sorts the list in ascending order based on the second element of each tuple.
# result of the previous code [(3, 1), (1, 2), (5, 4)]
6. Recursion
Recursion, also called recursivity, is a technique in which a function calls itself. It is used to solve problems that can be divided into smaller problems of the same kind. For example, the factorial of a number is the product of all the integers starting from 1 to that number. I.e., the factorial of 5 is 5 * 4 * 3 * 2 * 1 = 120.
Here is an example of a recursive function to calculate the factorial of a given number, here it is 5.
def factorial(n): print ("iteration number : " + str(n) ) if n == 1: return 1 else: return n * factorial(n-1) print(factorial(5))
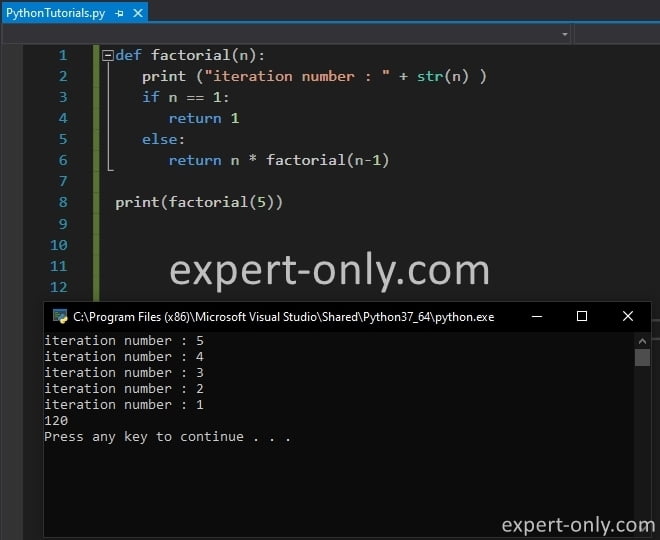
7. Conclusion on user-defined Python functions benefits
First, user-defined Python functions provide modularity to the code. You can define functions to perform specific tasks and then call those functions from other parts of your code, which makes your code easier to read and maintain. Functions also promote code reuse. You can define a function once and use it multiple times in your code, which saves you time and effort.
Functions in Python, or in any programming language in general, can help you write cleaner and more efficient code. By organizing the code into functions, it is easier to read, debug, and test. They also can improve the readability and understandability of the code, useful and even mandatory for a team of developers.
By giving descriptive names to your functions and organizing your code into logical blocks, you can make it easier for others to understand your code. Overall, using functions in Python can help you write better, more efficient, and more organized code.
To go further and learn about SSIS this time, here a step by step tutorial on how to import XML files into a SQL table using Integration Services.
Be the first to comment