Tutorial about the basic Python syntax with indentation, variables, loops and control structures code examples.
What are the basic Python syntax and how to use them in practical examples? Python is a popular programming language known for its simplicity, readability, and versatility. In this blog post, we will cover the basic syntax of Python, including indentation, variables, loops, and control structures.
To start this tutorial about basic programming syntaxes in Python, indentation is the first thing to talk about.
Table of Contents
1. Python indentation syntax
Indeed, in Python programming, indentation is used to indicate which lines of code belong to the same block. This is different from many other programming languages, which use curly braces to enclose blocks of code. For example, check the following code example, it does not use parenthesis to mark the end of if section, but the indentation.
if x > 0: print('x is positive') x += 1 print('x has been incremented') else: print('x is not positive')
In this code, the lines print('x is positive')
and x += 1
are both indented, indicating that they belong to the same block of code that is executed if x
is positive. The line print('x has been incremented')
is also indented, indicating that it belongs to the same block of code as the first two lines.
It is important to maintain consistent indentation throughout the code, as incorrect indentation can lead to syntax errors. Here are more examples to illustrate how indentation is used in Python code.
# Example 1 x = 8 if x > 0: print('x is positive') x += 1 else: print('x is not positive') # Example 2 for i in range(5): print(i) if i % 2 == 0: print('i is even') # Example 3 def welcome(name): print('Hello, welcome ' + name + '!') name ='expert' print( welcome(name) )
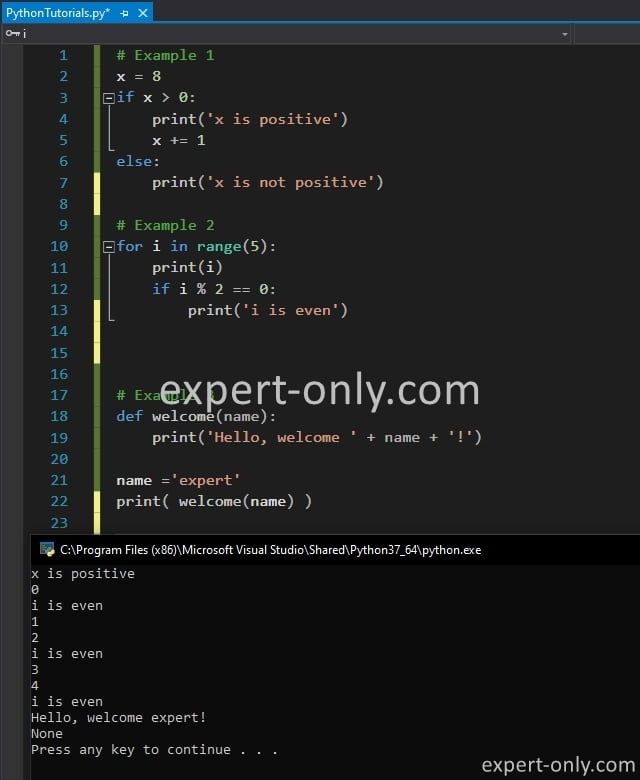
Another basic but fundamental element of the coding learning curve is the built-in data types available in the Python language.
2. Python basic syntax for variables
To store data in Python, you can use variables. A variable is a named location in memory where you can store a value.
2.1 Python variables assignement
To create a variable, you just need to give it a name and assign it a value. For example, this is a simple code example to create and print 3 variables with 3 different Python data types:
- Integer
- String
- Boolean
# Simple assignment of a single value to a variable x = 5 print(x) # Output: 5 # Assignment of a string value to a variable message = 'Hello, world!' print(message) # Output: 'Hello, world!' # Assignment of a Boolean value to a variable flag = True print(flag) # Output: True
2.2 Python variables are untyped
In Python, variables are untyped, meaning that you can assign a value of any data type to a variable without explicitly declaring its type. So it is possible to assign a value of a data type to a variable of a different data type. This is in contrast to languages like C or Java, where you must specify the type of a variable when you declare it.
For example, assign a varchar to an integer variable, and then a Boolean value.
# Assignment of an integer value to a variable x = 5 print(x) # Output: 5 # Reassignment of a string value to the same variable x = 'Hello, world!' print(x) # Output: 'Hello, world!' # Reassignment of a Boolean value to the same variable x = True
The advantage of untyped variables is that they can be more flexible and easier to use. However, it is important to be aware of the data type of a variable when you are working with it, as certain operations may only be valid for certain data types. For example, you cannot concatenate an integer and a string in Python, as the +
operator is used for both addition and concatenation.
x = 5 y = 'hello' # This will raise a TypeError z = x + y
To avoid this type of error, you can use type coercion to explicitly convert a value to a different data type.
x = 5 y = 'hello' # This will concatenate the string representation of x and y z = str(x) + y # the output will be : 5hello
You can use variables to perform operations and store the result. For example:
# Addition of two variables and assign the result to a new variable : z x = 5 y = 3 z = x + y print(z) # Output: 8 # Concatenation of two string variables and assign the result to c a = 'Hello, ' b = 'world!' c = a + b print(c) # The output is : 'Hello, world!'
2.3 More complex examples with Python variables
You can also use variables to store the result of a function call. Like in the following code example.
# Call to the len() function to get the length of a string # and assign the result to a variable word = 'hello' length = len(word) print(length) # Output: 5 # Call to the sum() function to add the elements of a list # and assign the result to a variable numbers = [1, 2, 3, 4, 5] sum = sum(numbers) print(sum) # Output: 15
Finally, you can use variables to store the result of a more complex expression. In the second code exemple, the variable is used to store the result of a function that checks if the string is a palindrome. I.e., words or phrases that are spelled the same way forwards and backwards, like :
- level
- madam
- rotator
# Calculation of the average of a list of numbers # then assign the result to the average variable numbers = [1, 2, 3, 4, 5] average = sum(numbers) / len(numbers) print(average) # Output: 3.0 # Check if a string is a palindrome (i.e., reads the same backward as forward) # then assign the result to the variable called is_palindrome word = 'racecar' is_palindrome = word == word[::-1] print(is_palindrome) # Output: True
3. Python loops
To continue and discover more on the Basic Python syntax, there are two types of loops in Python, the for loops and the while loops. As you can see in the code below, loops are a powerful tool in Python that can be used to perform repetitive tasks and process data.
3.1 For loop in Python
A for
loop is used to iterate over a sequence of elements, such as a list or a string. For example:
# Simple for loop example for i in range(5): print(i) # Output: 0 1 2 3 4 # For loop with a list colors = ['red', 'green', 'blue'] for color in colors: print(color) # Output: red green blue # For loop with a string word = 'hello' for letter in word: print(letter) # Output: h e l l o
3.2 While loop in Python
A while
loop is used to repeat a block of code as long as a certain condition is true.
# Simple while loop example i = 0 while i < 5: print(i) i += 1 # Output: 0 1 2 3 4 # While loop with a counter counter = 0 while True: print('Hello, world!') counter += 1 if counter == 5: break # Output: Hello, world! Hello, world! Hello, world! Hello, world! Hello, world! # While loop with user input while True: input_text = input('Enter a number: ') if input_text.isdigit(): input_number = int(input_text) print('You entered the number', input_number) break else: print('Invalid input, please try again.')
3.3 The break and continue statements
You can also use the break and continue statements within a loop to control its execution. The break statement will exit the loop prematurely, while the continue statement will skip the rest of the current iteration and move on to the next one.
First examples using break continue in a for loop
# For loop with a break statement for i in range(10): if i == 5: break print(i) # Output: 0 1 2 3 4 # For loop with a continue statement for i in range(10): if i % 2 == 0: continue print(i) # Output: 1 3 5 7 9
Examples using break continue in a while loop
# While loop with a break statement i = 0 while True: print(i) i += 1 if i == 5: break # Output: 0 1 2 3 4 # While loop with a continue statement i = 0 while True: i += 1 if i % 2 == 0: continue print(i) if i == 10: break # Output: 1 3 5 7 9
4. Syntax of basic control structures
Control structures are used to control the flow of execution of a program. In Python, there are three control structures: if
, elif
, and else
.
4.1 If statement in Python
An if
statement is used to execute a block of code if a certain condition is true.
x = 5 if x > 0: print('x is positive') # Output: x is positive
4.2 If else statement
You can also use an if
statement in conjunction with an else
clause to execute a different block of code if the condition is false.
x = 5 if x > 0: print('x is positive') else: print('x is not positive') # Output: x is positive
4.3 If elif else statement
If you have multiple conditions that you want to check, you can use an elif
clause to specify additional conditions.
x = 5 if x > 0: print('x is positive') elif x < 0: print('x is negative') else: print('x is zero') # Output: x is positive
5. Conclusion on Python syntax
In this programming tutorial, we covered the basic syntax of Python, including variables, loops, and control structures.
We also learned how to use variables to store and operate on data, and how to use loops and control structures to perform tasks and make decisions in our code. And about some of the unique features of Python, such as its use of indentation to indicate blocks of code and its support for untyped variables.
With these fundamentals, you can now start writing your own Python programs. Whether you want to build a simple script to automate a task, or a complex application to solve a real-world business problem, Python is a powerful and flexible language that can help you achieve your goals.
Popular IT tutorials that might interest you
- Calculate the last year value in Power BI with DAX
- Calculate the difference between two dates with SQL Server
- Insert or remove SQL Server line breaks from text
- Rename multiple Windows files at the same time
Be the first to comment