Tutorial on Python lists and how to create, append, modify, index, insert or delete elements with scripts examples.
Lists are a fundamental data structure in Python and understanding how to use them effectively is crucial for any Python developer. This tutorial covers the basics of lists, including creating and manipulating them, using list methods and comprehension, and working with multidimensional lists. Code examples and best practices are provided throughout the tutorial to help in understanding and implementing the concepts discussed.
Table of Contents
1. Introduction to Python lists
Python lists are a powerful and versatile data structure that allows you to store and manipulate a collection of items. They are enclosed in square brackets [] and can contain elements of any data type, such as numbers, strings, and even other lists. Lists in Python are mutable, meaning that you can add, remove, and change elements within the list.
Python lists are also ordered, so each element has a specific position within the list. Understanding how to work with lists in Python is an essential skill for any programmer, as they are commonly used in a wide range of applications. Below a script example to create 4 different types of lists:
- Numbers
- Strings
- A mixed list
- A list of lists
numbers = [1, 2, 3, 4, 5] words = ['Hello', 'World'] mixed = [1, 'Hello', 3.14] # a list of lists lists = [[1, 2, 3], [4, 5, 6]]
2. Lists indexing and slicing
Just like Python strings, lists can be indexed and sliced, allowing you to access individual items or sublists.
List indexing and slicing is a powerful feature in Python that allows you to access individual items or sublists within a list.
2.1 Index lists in Python
The basic syntax for indexing a list is to use square brackets [] and the index of the item you want to access. For example, to access the first item of a list called my_list, use the following code.
my_list = [1, 2, 3, 4] first_item = my_list[0] print(first_item) # the output is :[1]
2.2 Slice a list in Python
Slicing a list is like indexing, but instead of accessing a single item, you can access multiple items by specifying a range. The basic syntax for slicing a list is to use square brackets [] with a colon : between two indices. The first index is the starting position, and the second index is the ending position. Let’s say we want to access the items from the first to the third in a list called my_list, use the script below.
my_list = [1, 2, 3, 4] sub_list = my_list[0:3] print(sub_list) #
2.3 Negative indice in Python
It’s also possible to use negative indices in list indexing and slicing. A negative index refers to an item counting from the end of the list. To access the last item of the list, use the specific script below.
my_list = [1, 2, 3, 4] last_item = my_list[-1] print(last_item)
2.4 Slice a list with an offset
In addition, it’s also possible to slice a list with an offset different from 1 (also called step value). For example, if we want to access every other item in a list, we can use the following code. Indeed, the Python script only displays one value from the list every two.
my_list = [1, 2, 3, 4, 5, 6, 7] every_other_item = my_list[0:7:2] print(every_other_item) # displays : [1,3,5,7]
3. Lists methods in Python
Python provides several built-in methods to work with lists, including methods for adding, removing, and modifying elements. These methods allow you to easily manipulate and organize your data in a list. Some commonly used Python methods to manage lits are the following:
- Append: Adds an item to the end of the list.
- Extend: Adds multiple items to the end of the list.
- Insert: Inserts an item at a specific position in the list.
- Remove: removes the first occurrence of an item from the list.
- Pop: removes the last item from the list and returns it.
- Index: returns the index of the first occurrence of an item in the list.
- Count: returns the number of occurrences of an item in the list.
3.1 Python append method
The Python “append” method is a built-in function that allows you to add an item to the end of a list. And the basic syntax for the append method is illustrated in the 3 scripts below
- The first example adds the number 4 to the list.
- In the second example, if we have a list called “numbers” and we want to add the numbers 4 and 5 to the end of the list, we would use the following script.
- In the third example, let’s say we have a list of fruits called “fruits” and we want to add “mango” to the end of the list, we would use the few lines of code below.
# add one item at a time my_list = [1, 2, 3] my_list.append(4) print(my_list ) # add multiple items at a time numbers = [1, 2, 3] numbers.append(4) print(numbers) fruits = ["apple", "banana", "orange"] fruits.append("mango") print(fruits)
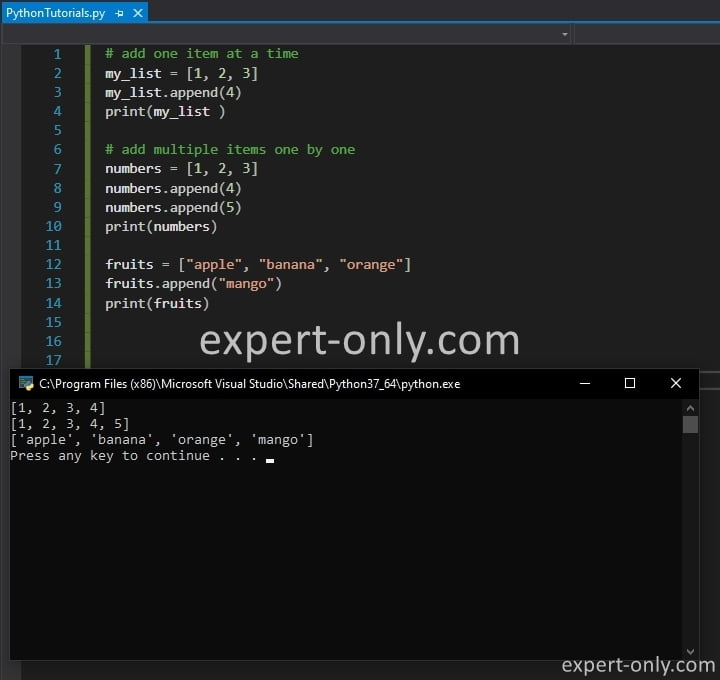
3.2 Extend method in Python
The extend()
method use with Python lists allows you to add multiple elements to a list. It takes an object than support iterations, such as a list or a tuple, as an argument and adds each element from that iterable to the original list. Here’s an example of using the extend()
method.
# Initial list original_list = [1, 2, 3] # List to be added new_elements = [4, 5, 6] # Using extend() to add new elements original_list.extend(new_elements) print(original_list) # Output: [1, 2, 3, 4, 5, 6]
The extend()
method in Python allows you to add multiple elements to a list. It takes an iterable object, such as a list or a tuple, as an argument and adds each element from that iterable to the original list. Here’s an example of using the extend()
method with lists containing numbers.
# Initial list original_list = [1, 2, 3] # List to be added new_elements = [4, 5, 6] # Using extend() to add new elements original_list.extend(new_elements) print(original_list) # Output: [1, 2, 3, 4, 5, 6]
As you can see, the extend()
method has added the elements from the new_elements
list to the original_list
. Another example, this time with lists of words.
# Initial list original_list = ['apple', 'banana', 'cherry'] # Tuple to be added new_elements = ('date', 'elderberry') # Using extend() to add new elements original_list.extend(new_elements) print(original_list) # Output: ['apple', 'banana', 'cherry', 'date', 'elderberry']
As you can see, the extend()
method can be used to add elements of any iterable object to a list. It is important to note that the extend()
method modifies the original list in place, meaning that it doesn’t return a new list but modifies the original list. If you want to create a new list with the added elements, you can use the addition (+) operator to concatenate the original list with the new elements.
3.3 Python insert method
The insert()
method in Python is used to insert an element at a specific position in a list. The syntax for the insert method is as follows, in the first example.
Index is the position where the element should be inserted, and element
is the value to be inserted. ere is an example of how to use the insert()
method to add the value cherry at the third position of a list of fruits.
list.insert(index, element) fruits = ["apple", "banana", "orange"] fruits.insert(2, "cherry") print(fruits) # output: ["apple", "banana", "cherry", "orange"]
As you can see in the script above, the insert()
method can be used to add an element at a specific position in a list. The index starts from 0.
3.4 Python remove method
element
is the value to be removed from the list. If the specified element is not found in the list, a ValueError
is raised. In the second example below is on how to use the remove()
method to remove the value “banana” from a list of fruits.
#generic syntax list.remove(element) fruits = ["apple", "banana", "orange"] fruits.remove("banana") print(fruits) # the output is :["apple", "orange"]
As you can see, the “banana” has been removed from the list. You can also use the Python del statement to remove an element by its index position.
fruits = ["apple", "banana", "orange"] del fruits[1] print(fruits) # the output is :["apple", "orange"]
3.5 Python lists pop method
The remove()
method in Python is used to remove the first occurrence of a specific value from a list. The syntax to use the remove()
Python method is like in the script below, it removes the number 3 from the list and displays it using the print function.
numbers = [1, 2, 3] last_number = numbers.pop() print(last_number) # displays :3
3.6 Python lists index method
The index()
method in Python is used to find the index of the first occurrence of a given item in a list. The item to be searched is passed as an argument to the method. If the item is not found in the list, a ValueError
is raised.
Here is an example of using the index()
method to find the index of an item in a list.
my_list = [1, 2, 3, 4, 5] my_list.index(3) # result:2
3.7 Python lists count method
The count()
method in Python is used to find the number of occurrences of a given item in a list. The item to be searched is passed as an argument to the method. If the item is not found in the list, it will return 0.
Here is an example of using the count()
method to find the number of occurrences of an item in a list. This simple Python script counts the number of occurrences of the number 3, i.e., 2.
my_list = [1, 2, 3, 3, 4, 5, 6, 7, 7, 8] my_list.count(3) 2
These selects examples are just a few amongst the many built-in methods available for working with lists in Python.
4. List comprehension
List comprehension is a concise way to create a new list from an existing list. It’s a way to transform one list into another list, or to create new Python lists that meets certain criteria.
For example, here’s a way to use list comprehension to create a new list of the squares of the numbers in an existing list.
numbers = [1, 2, 3, 4, 5] squares = [num ** 2 for num in numbers] print(squares) # result: [1, 4, 9, 16, 25]
You can also use conditionals in list comprehension to filter the items in the list. For example, here’s how to use list comprehension to create a new list of even numbers in an existing list. The Python script uses the modulo function.
numbers = [1, 2, 3, 4, 5] even_numbers = [num for num in numbers if num % 2 == 0] print(even_numbers) # prints: [2, 4]
In the next chapter of the tutorial about multidimensional lists, we’ll use the Python modules concepts to retrieve data in specific positions from a 2D matrix.
5. Multidimensional lists in Python
Python also supports Multidimensional Lists (lists of lists). They can be created by nesting lists, i.e., list of lists. For example, a 2D matrix could be represented as a list of lists.
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] print(matrix[0][0]) # 1 print(matrix[2][2]) # 9
You can also use list comprehension for multidimensional lists. For example, you can use a nested list comprehension to extract a specific column from a matrix. The following matrix has 3 rows and 3 columns. The scripts below written in Python displays the second element of each row of the matrix.
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] column = [row[1] for row in matrix] print(column) # prints: [2, 5, 8]
A matrix is a rectangular array of numbers, symbols, or expressions, arranged in rows and columns. Matrices are often used to represent and manipulate large sets of data, such as in linear algebra, physics, and computer graphics.
In IT, matrices are used to represent arrays and are often used in data structures like 2D arrays and 3D arrays. They are also used in image processing and computer graphics to represent images and other visual data.
6. Conclusion on using the Python lists
In summary, lists in Python are a powerful tool for organizing and manipulating data. They can be used for various operations such as appending, extending, inserting, and removing items. Additionally, list comprehension is a useful feature for creating new lists in a concise and readable way, and filtering items based on conditions. Python also supports multidimensional lists, which can be useful in mathematical, scientific, and game development applications. With practice, you can use lists to build complex and dynamic programs.
In most of the IT projects, scripting is used along side with SQL, here a tutorial with the list of all the T-SQL data types.
Be the first to comment