How to use Python strings and dedicated functions like concatenate, index, slice and format texts?
Working with strings in Python is a very powerful tool and a long journey. It is one of the most used and important data types in any programming language. Strings are everywhere, from simple messages to complex data formats. This data type is used to display information, to store data, to connect to web services, and many more. Mastering the art of strings in Python, will give you the ability to create more dynamic and interactive programs. And to manage characters while developing in a more efficient way.
In this tutorial, you will uncover some of the secrets of strings in Python. And you’ll learn how to create, manipulate, format, and perform operations on them. You will learn how to use different string formatting techniques like the percentage operator, the format method, and the f-strings.
Additionally, you will see some examples of the most used string operations such as concatenation, repetition, and membership testing. By the end of this article, you will have a solid understanding of how to work with strings in Python, and you’ll be able to unleash your creativity and build something powerful.
Table of Contents
1. Introduction to Python strings
Strings in Python are a sequence of characters, including letters, numbers, and symbols, that can be used to represent any kind of textual data. In Python, strings are enclosed in single or double quotes, and can be easily created and manipulated using a variety of built-in functions and methods.
Some of the most common operations used with strings include concatenation, which allows you to combine multiple strings together, slicing, which allows you to extract specific characters or substrings from a string, and string formatting, which allows you to insert variables into a string and control their placement.
Indeed, a string is a sequence of characters, such as letters, numbers, and symbols. For example, this Python script example creates 2 strings which contains the famous Hello, World! sentence.
string1 = 'Hello, World!' string2 = "Hello, World!"
1.1 Simple and double quotes difference
Both string1
and string2
are equivalent for classical cases, but different. Indeed, in Python, the difference between single quotes and double quotes is that they are both used to define a string, but they handle certain characters differently.
Single quotes (‘ ‘) are used to define a string that contains no single quotes, while double quotes (” “) are used to define a string that contains no double quotes. For example, if you want to create a string that contains the single quote character, you would use double quotes to define the string, and if you want to create a string that contains the double quote character, use single quotes to define the string.
Another difference is that string literals enclosed in single quotes are slightly faster when compared to double quotes. Both the above statements will create the same string, but the first one is enclosed in single quotes and the second one in double quotes.
In summary, you can use either single or double quotes to define a string in Python, but if the string contains a quote of the same type as the enclosing quotes, you will need to use the opposite type of quotes.
1.2 Escape character in Python strings
In python, you can use the backslash () character as an escape character to include special characters, such as quotes, in a string. The backslash tells Python to treat the next character as a literal, rather than as a special character.
# using escape character for double quote string1 = "This is a string with a \"quote\" inside it" # using escape character for single quote string2 = 'This is a string with a single quote (\') inside it'
You can also use the backslash to include newlines, tabs, and other special characters in the string. It’s also worth noting that you can use raw strings. Prefix the string with ‘r’ or ‘R’ , this will tell Python to ignore any escape character in the string.
# using escape character for newline string3 = "This is a string with a newline character at the end of it\n" # using escape character for tab string4 = "This is a string with a tab character at the end of it\t" # Using a raw string string5 = r"This is a string with a newline character at the end of it\n"
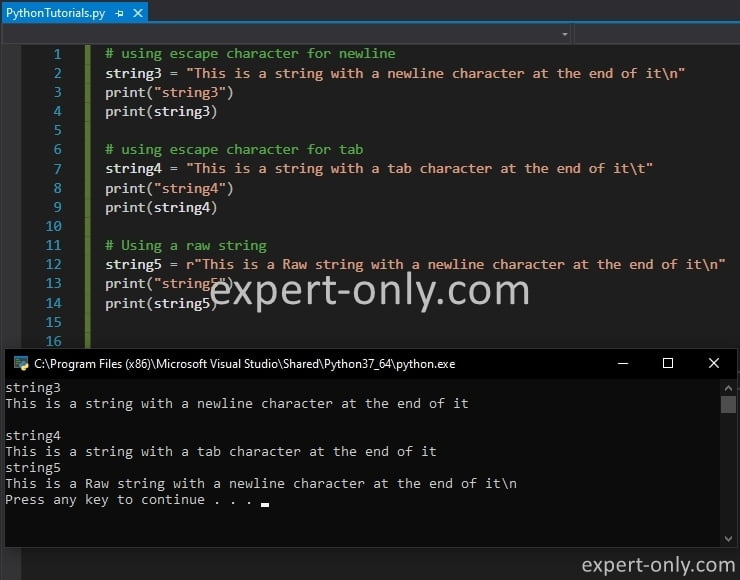
In summary, the backslash character is used to escape special characters, such as quotes and newlines, in a string in Python. This allows you to include these characters within a string, rather than treating them as special. To work with the other data types, follow this tutorial with list of the most used Python data types and scripts examples.
2. String concatenation
In Python, you can concatenate two strings using the +
operator. For example, this script executes a simple concatenation of two strings.
string1 = 'Hello' string2 = 'World' result = string1 + ', ' + string2 print(result)
3. How to index Python strings?
You can access individual characters in a string using indexing. In Python, strings are indexed starting from 0. For example:
string = 'Hello, World' print(string[0]) # H print(string[7]) # W
You can also use negative indexing to access characters from the end of the string. For example:
print(string[-1]) # d print(string[-5]) # o
4. How to cut Python strings?
You can extract a specific section of a string using slicing. You notice here that the Python syntax is similar to one used for the list data type.
string = 'Hello, World' print(string[7:12]) # World print(string[:5]) # Hello print(string[7:]) # World
Python is widely used to manipulate data and makes it a powerful tool in analytics projects, it is often used in addition with other tools like Power BI and its DAX language. This tutorial helps to calculate the value of the previous month in DAX.
5. String methods in Python
Python strings have several built-in methods that allow you to perform various operations. Some of the most common string methods include the following, but not exhaustive, list.
- upper: converts the string to uppercase.
- lower: converts the string to lowercase.
- strip: removes whitespaces from the beginning and end of the string.
- replace: replaces all occurrences of the old string with the new string.
- split: splits the string into a list of substrings, using the specified separator.
- find: returns the index of the first occurrence of the specified substring, or -1 if the substring is not found.
5.1 Python strings upper function
The upper()
function in Python is a built-in method that can be used to convert all the characters in a string to uppercase. The function takes no arguments and returns a new string with all the characters in uppercase.
original_string = "Hello World" uppercase_string = original_string.upper() # will print "Hello World" print(original_string) # will print "HELLO WORLD" print(uppercase_string)
Another use case is when you want to make sure that all the characters in a string are in uppercase, for example, when you want to display text in uppercase letters. Then you can use the upper function to simply compare the upper result with the original string.
5.2 Python strings lower function
The lower()
function in Python is a built-in method that can be used to convert all the characters in a string to lowercase. The function takes no arguments and returns a new string with all the characters in lowercase.
original_string = "Hello World" lowercase_string = original_string.lower() # will print "Hello World" print(original_string) will print "hello world" print(lowercase_string) #
5.3 String strip functions
The strip()
function in Python is a built-in method that can be used to remove leading and trailing white spaces from a string. And this function takes no arguments and returns a new string with the leading and trailing white spaces removed. The white spaces include spaces, tabs and newline characters.
original_string = " Hello World " stripped_string = original_string.strip() print(stripped_string)
Other similar functions are lstrip()
and rstrip()
, they are used to remove leading and trailing white spaces respectively.
5.4 String replace function
The replace()
function in Python is a built-in method that can be used to replace a specific substring within a string with another substring. The function takes two arguments: the substring to be replaced, and the substring to replace it with. It returns a new string with the specified replacements made.
original_string = "Hello World" replaced_string = original_string.replace("World","Python") # prints Hello Python print(replaced_string)
It’s worth knowing that the Python replace function does not modify the original string, but only returns a new one with the specified replacements made. Unless you write the string in the original string variable of course.
5.5 String split function
The split()
function in Python is an incredibly powerful and versatile method for breaking up strings into smaller substrings! This function allows you to split a string into a list of substrings based on a specified delimiter. The delimiter can be any character or sequence of characters that you specify.
For example, let’s say you have a string that contains a list of items separated by commas, and you want to turn that string into a list of individual items. You can use the split()
function to accomplish this!
# example using a comma as a delimiter original_string = "apple,banana,orange,grape" list_of_fruits = original_string.split(",") print(list_of_fruits) # example with a space delimiter original_string = "This is an example" list_of_words = original_string.split(" ") print(list_of_words)
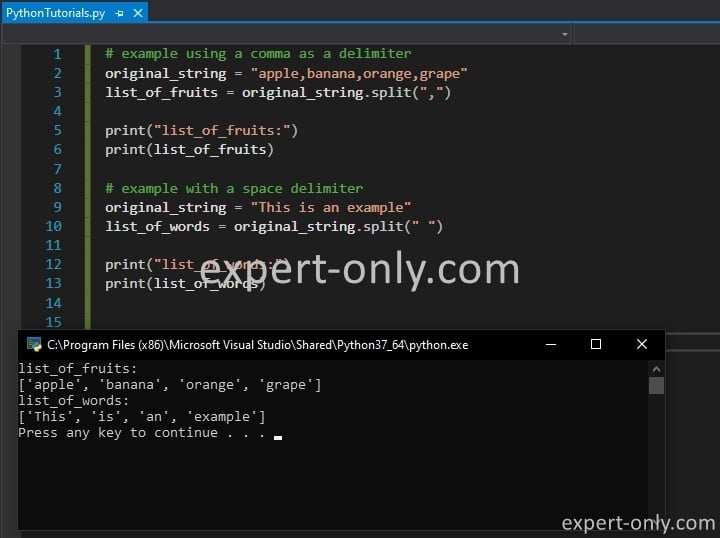
The second example in the code above will output the list of words [‘This’, ‘is’, ‘an’, ‘example’]. The function split()
uses the whitespace as the delimiter to split the string into a list of substrings.
5.6 String find function
The find()
function in Python is a built-in method that can be used to locate the first occurrence of a substring within a string. The function takes one argument, the substring you want to find, and returns the index of the first occurrence of the substring within the string. If the substring is not found, the function returns -1.
The following script prints the integer 6, which is the index of the first occurrence of the substring “World” in the string “Hello World”.
Note that the find function in Python starts counting at 0.
original_string = "Hello World" index = original_string.find("World") print(index)
In addition to all the built-in functions available in Python, it is also possible to built your own user-defined Python functions dedicated to a specific task that is used multiple times in a program.
6. String formatting
String formatting is the process of creating a new string by replacing placeholders in an existing string with actual values. Python provides several ways to format strings. The most basic way is to use the percentage (%) operator, also known as the string formatting operator. For example:
name = "John" age = 20 string = "My name is %s and I am %d years old" % (name, age) print(string) # My name is John and I am 20 years old
Another way to format strings is by using the format()
method. This method allows you to define placeholders in the string, and then replace them with actual values using the method’s arguments. For example:
name = "John" age = 20 string = "My name is {} and I am {} years old".format(name, age) print(string) # My name is John and I am 20 years old
Python f-string function
In python 3.6 and the above versions, you could use the f-strings function (also called f-literals) which is the most elegant and efficient way to format strings. For example:
name = "John" age = 20 string = f"My name is {name} and I am {age} years old" print(string) # My name is John and I am 20 years old
7. String operations in Python
Another way to work with strings is to use different operations, such as concatenation, repetition, and membership testing. For example:
string1 = 'Hello' string2 = "World"
7.1 Concatenation
Concatenation is the process of combining two or more strings together to form a single string. In Python, there are several ways to concatenate strings, including using the +
operator, the *
operator and the join()
method.
The most basic way to concatenate strings is to use the +
operator. For example, to concatenate the strings “Hello” and “World”, you would use the following code:
string1 = "Hello" string2 = "World" concatenated_string = string1 + " " + string2 print(concatenated_string) # result is :Hello World
7.2 Python string repetition using the star operator
Another way to concatenate strings is to use the *
operator. This operator allows you to repeat a string a certain number of times. For example, to concatenate the string “Hello” three times, you would use the following code.
string = "Hello" concatenated_string = string * 3 print(concatenated_string) # result is :HelloHelloHello
7.3 Python join method
The join()
method is used to concatenate a list of strings with a specified delimiter. For example, to concatenate a list of strings with a comma as the delimiter, you can use the following script and adapt it to your specific use case of course.
list_of_strings = ["Hello", "World", "!"] concatenated_string = ",".join(list_of_strings) print(concatenated_string) # prints :Hello,World,!
7.4 Membership testing
print('H' in string1) # True print('W' in string1) # False
These are just a few examples of the various string operations that are available in Python. It’s worth noting that strings are immutable, meaning that their value cannot be changed after they are created. However, you can create a new string by combining or manipulating the original string in various ways.
In this article we covered a lot of the basic and most used functionalities of Python Strings and Lists. It’s just the tip of the iceberg, but with this foundation you will be able to tackle more complex problems and write more powerful code. Always keep in mind that practice makes perfect. Happy coding!
8. Conclusion on working with Python strings
To conclude, working with strings in Python is a crucial aspect of any software development. Python provides several ways to format strings such as using the %
operator, the format()
method and f-strings, allowing you to replace placeholders in an existing string with actual values. These are very useful when building applications, to display data in a user-friendly way, but also to create strings that match specific patterns.
Furthermore, you’ve also learned how to use different operations on strings such as concatenation, repetition, but also the membership tests. You should now have a solid understanding of how to manipulate strings in Python programming. And you can apply this knowledge to many different areas of programming. Remember that practice makes perfect. So the more you work with strings, the better you’ll get at using them in your projects.
Be the first to comment