Python tutorial on how to manage text files using the open, read, readline, write, writelines, seek and close methods with scripts examples.
This tutorial on how to manage text files in Python provide some information and code examples on working with files using Python scripts. Text files are a fundamental part of computer science and are used to store plain text data. They are widely used in various applications, such as storing configuration files, logs, and data for machine learning models. Python programming allows to easily read, write, append and manipulate lines using the built-in functions and modules.
Table of Contents
1. File opening modes in Python
In order to manage text files using Python scripts, you must know the file access modes and their specificities. The file access modes in Python are used to determine the type of operations that can be performed on files after opening them. These modes also determine the location of the Python reading method, it is like a cursor, called file handle. It is important because it defines the position of reading and writing, physically in the file system.
There are six different file access modes in Python that are used to open and manipulate files.
# | File opening mode name | Syntax |
---|---|---|
1 | Read only mode | r |
2 | Read and write only mode | r+ |
3 | Write only mode | w |
4 | Write and read | w+ |
5 | Append only | a |
6 | Append and read | a+ |
1. Read only mode: r
When working with files with Python scripts, the read only mode, or r mode is useful to open a text file for reading only. This means that the file can be read, but not written to. When a file is opened in this mode, the file pointer is set at the start of the file. In case the file specified does not exist, it will result in an input/output error. The read only mode is the default mode in which a file is opened in absence of any specified mode.
2. Read and write only mode: r+
The read and write mode, also called the r+ mode in Python file handling is used to open a file for both reading and writing operations. This means that once the file is opened in this mode, you can read from it as well as write to it.
The file pointer is positioned at the start of the file, so you can begin reading or writing from the very beginning. However, if the specified file does not exist, it will result in an Input/Output error (also called I/O error), as no operations can be performed. This mode is useful when you want to both read from and write to a file, while keeping the file pointer at the beginning of the file.
3. Write only mode: w
The w mode, also called the write only mode in Python file management is used to open a file exclusively for writing operations. This means that once the file is opened in this mode, you can write to it, but not read from it. If the file already exists, all the data in the file is truncated and overwritten. In this mode, the file pointer is also positioned at the beginning of the file.
In case the specified file does not exist, a new file will be created with the same name. This mode is useful when you want to create a new file or overwrite an existing file. It is important to note that, it’s a dangerous mode to use as it may lose the data if the file already exists, so it’s good to have a backup before using it. It also special because you cannot check the content of files before writing.
4. Write and read mode: w+
The Python w+ mode is used to open a file for both reading and writing operations. This means that once the file is opened in this mode, you can read from it as well as write to it.
However, if the file already exists, all the data in the file will be truncated and overwritten. Indeed, any existing content in the file will be deleted and replaced with the new data. Also, if the specified file does not exist, a new file will be created with the same name. This mode is useful when you want to create a new file or overwrite an existing one while being able also to read it afterwards.
5. Append only mode: a
The append mode in Python, is used to open a file exclusively for writing operations, but with a specific feature, the file handle is positioned at the end of the file. Technically, it means that any data written to the file will be appended to the end of the existing data.
If the specified file does not exist, a new file will is created with the name provided. This mode is very useful to add new data to an existing file without overwriting any of the existing data. It’s worth noting that, the file is created if it does not exist, so it’s a safe option to use when you want to add new data to a file without losing the existing one. This mode is particularly useful with log files, to keep track of new events without erasing previous records.
6. Append and read mode: a+
The append and read mode in Python, is useful to open a file for read and also write operations. It simply the read option the append only mode. Like in the previous mode wee saw, with the a+ mode, the file handle is positioned at the end of the file, i.e., the data written is inserted after the existing data.
This mode is useful when you want to append new data to an existing file, while still being able to read the contents of the file afterwards, for example, to read the last line written in the file. Also in this mode, any existing data in the file is not overwritten, only new data is added at the end of the file.
2. How to manage text files with basic Python operations?
In Python, working with text files involves opening the file, performing operations on it, and closing the file. Here are some basic operations that can be performed on text files in Python using the open, read, write and close methods.
2.1 Opening a text file in Python
To open a text file in Python, we use the built-in open()
function. The function takes the file name and the mode in which the file should be opened (e.g. ‘r’ for reading, ‘w’ for writing, ‘a’ for appending, etc.) as arguments. Here is a first example of how to open a text file in read-only mode.
file = open('example.txt', 'r')
2.2 Read a text file in Python
Once a file is opened, it can be read using the read()
method. This method takes an optional argument that specifies the number of characters to be read. If no argument is passed, the entire file is read. Here is a second example, this time on how to read the entire contents of a file.
content = file.read() print(content)
2.3 Write to a text file in Python
To write to a text file, it must be opened in write mode (‘w’). The write()
method is used to write data to the file. Here is the third example of the section, with a script to write a string to a text file.
file = open('example.txt', 'w') file.write('Hello World')
2.4 Close a text file using Python
It is important to close a file after performing operations on it to ensure that the changes are saved and to release the resources used by the file. The close()
method is used to close a file.
file.close()
It’s important to note that when you open a file in write mode, the existing content of the file is truncated, so the data in the file is deleted, and new data is written. To go further on Python programming concepts, the best way to manage lines from files is to use the Python lists data type.
In the next sections, we will cover more advanced Python operations with text files such as reading and writing in specific positions, appending, and manipulating the content of text files.
3. Read text files with Python
In this second section about how to manage files in Python, we will delve deeper into reading text files in Python. We will cover different techniques for opening and reading text files, including reading a specific number of characters or lines, iterating over the lines of a text file, and storing the contents of a text file in a list or other data structure. We will also provide examples and code snippets to demonstrate each technique in action.
3.1 Open and read a text file in Python
As mentioned in the introduction, the open()
function is used to open a text file in Python. To read a text file, it should be opened in read mode (‘r’). Once the file is open, the entire contents of the file can be read using the read()
method. Here is an example of how to open a text file, again in read only mode and read its contents, using 4 lines of scripts.
In this example, the script reads the file located in C:\data\example.txt in read only mode and display the full content, i.e., 21 lines of data. The file includes 1 header line and 20 lines of data.
file = open('C:\data\example.txt', 'r') content = file.read() print(content) file.close()
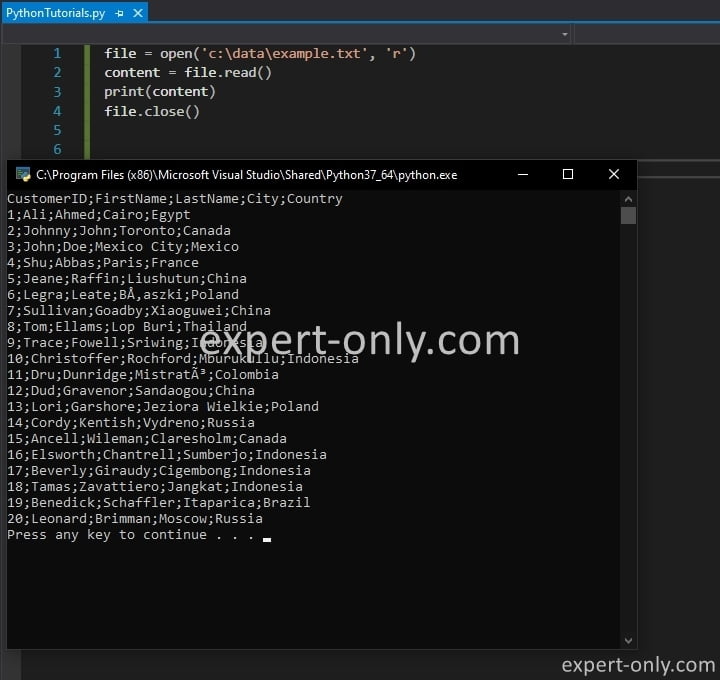
3.2 Read a specific number of characters or lines
This second example of the section on reading text files using Python is a little bit more advanced. Indeed, sometimes, we only need to read a specific number of characters or lines from a text file. To do so, we use two different methods, often used together:
- The
read()
method take an optional argument that specifies the number of characters to read. - The
readline()
method is used to read a single line of a text file.
Here is a practical example of how to read only the first 12 characters and the first line of a text file.
file = open('example.txt', 'r') ten_chars = file.read(12) print(ten_chars) first_line = file.readline() print(first_line) file.close()
3.3 Iterate over the lines of a text file in Python
The readline()
method we just saw above can of course be used in a loop to iterate over the lines of a text file. This is the more efficient way of reading a text file when compared to reading the entire file into memory.
Below is another example of Python script, it iterates over all the lines of the text file called example.txt.
file = open('example.txt', 'r') for line in file: print(line) file.close()
3.4 Read a file line by line and store it in a list with Python
We can also read a text file line by line and store the data in a list or any other suitable Python data structure. The Python script below reads a text file line by line and store it in a list, called lines. Note that it is possible and recommended for long term storage, to use a SQL database.
file = open('example.txt', 'r') lines = file.readlines() print(lines) file.close()
Please note that the Python programming language provide an elegant way to handle file using the context manager. The context manager takes care of opening and closing the file automatically. I.e., In this example, you don’t have to explicitly close the file.
with open("example.txt", "r") as file: content = file.read() print(content)
In the examples above, we’ve used the .txt
file, but you can read any other file format as well, such as .csv
, .json
, etc.
4. Write text files with Python scripts
In the following sections, we will cover more advanced operations such as writing to a text file and manipulating the contents of a text file.
4.1 Open and write to a text file in Python
In Python, the open()
function is used to open a file and the write()
method is used to write to a file. The first argument of the open()
function is the path to the file and the second argument is the mode in which the file should be opened. The mode 'w'
is used to open a file for writing, and truncates the file if it already exists. Here’s an example of how to open and write to a text file:
with open('example.txt', 'w') as file: file.write('Hello World')
In the script above, the file example.txt
is opened in write mode and the string 'Hello World'
is written to the file. The with open
statement is used to ensure that the file is properly closed after the operations inside the block are completed.
4.2 Write to a specific position in a text file
The seek()
method can be used to move the file pointer to a specific position in a file. The first argument of the seek()
method is the position and the second argument is an optional value that defaults to 0
. Here’s an example of how to write to a specific position in a file, here it is the position 6, i.e., just after the word Hello and the space written just before.
with open('example.txt', 'w') as file: file.write('Hello ') file.seek(6) file.write('Universe')
In this example, the file example.txt
is opened in write mode and the string 'Hello '
is written to the file. Then the file pointer is moved to position 6 using the seek()
method and the string 'Universe'
is written to the file. This will change the text from ‘Hello ‘ to ‘Hello Universe’
4.3 Append text to a file with Python
The mode 'a'
is used to open a file for appending. In this mode, any data written to the file is automatically appended to the end of the file. Here’s an example of how to append text to a file.
with open('example.txt', 'a') as file: file.write('Hello World')
In this example, the file example.txt
is opened in append mode and the string 'Hello World'
is written to the file.
4.4 Write to a file line by line
The writelines()
method can be used to write a list of strings to a file, with each string as a separate line. Here’s an example of how to write to a file line by line:
lines = ['Hello', 'World'] with open('example.txt', 'w') as file: file.writelines('\n'.join(lines))
In this example, the list lines
is created and contains two string elements 'Hello'
and 'World'
. Then the file example.txt
is opened in write mode and the writelines()
method is used to write the elements of the list to the file as separate lines.
It is important to remember that when you are working with text files, it is recommended to always use the with open
statement to ensure that the file is properly closed after the operations inside the block are completed.
5. Manipulate data in text files using Python
In this fourth section on how to manage files in Python, let’s see how to use Python scripts to manipulate text files. We will cover various operations such as searching for a specific string or pattern, replacing text, sorting lines, and extracting specific lines. Detailed explanations, methods and code examples will be provided for each use case to help you understand the process and apply it to your own projects.
5.1 Search for a specific string using the find method
Searching for a specific string in a text file can be done using the str.find()
method or the re
module for regular expressions. The str.find()
method returns the index of the first occurrence of the specified string in the text file. If the specified string is not found, it returns -1. This script uses the str.find()
Python method to search for a specific string in a text file.
with open("example.txt", "r") as file: text = file.read() index = text.find("example") if index != -1: print("The string 'example' was found at index", index) else: print("The string 'example' was not found in the text file.")
5.2 Search specific strings using search method
The re
module can also be used to search for a specific string by using the search()
function. The search()
function returns a match object if a match is found and None
if no match is found. Here is an script to show how to use the search()
function to search for a specific string in a txt file.
import re with open("example.txt", "r") as file: text = file.read() match = re.search("example", text) if match: print("The string 'example' was found at index", match.start()) else: print("The string 'example' was not found in the text file.")
5.3 Search for a specific string with Python
Searching for a specific string in a text file can be done using the str.find()
method or the re
module for regular expressions. The str.find()
method returns the index of the first occurrence of the specified string in the text file. If the specified string is not found, it returns -1. Find another way to search for a string inside a text file using, this time with the str.find()
method.
with open("example.txt", "r") as file: text = file.read() index = text.find("example") if index != -1: print("The string 'example' was found at index", index) else: print("The string 'example' was not found in the text file.")
The re
module can also be used to search for a specific string by using the search()
function. The search()
function returns a match object if a match is found and None
if no match is found. Use the search()
function to search for a specific string in a txt file, as in the code below.
import re with open("example.txt", "r") as file: text = file.read() match = re.search("example", text) if match: print("The string 'example' was found at index", match.start()) else: print("The string 'example' was not found in the text file.")
5.4 Search patterns with findall and finditer
Searching for a specific pattern in a text file can be done using the re
module. The findall()
function returns a list of all the matches in the text file. One more example of how to use the findall()
function to search for a specific pattern in a text file.
import re with open("example.txt", "r") as file: text = file.read() matches = re.findall("[0-9]+", text) print("The following numbers were found in the text file:", matches)
The finditer()
function can also be used to search for a specific pattern in a text file. It returns an iterator yielding match objects.
import re with open("example.txt", "r") as file: text = file.read() for match in re.finditer("[A-Za-z]+", text): print("The word", match.group(), "was found at index", match.start())
5.5 Replace a text in a file in Python
To replace text in a file in Python, use the replace()
method. This method replaces all occurrences of the specified string with another string. Here is another script to illustrate how to use the replace()
method to replace text in a text file.
It is important to note that when using the replace()
method, the original file will be overwritten with the new text. Indeed, to keep the original file, create a backup copy or create new file and write the new text there.
with open("example.txt", "w") as file: new_text = text.replace("old_text", "new_text") file.write(new_text) print("Text replaced successfully.")
5.6 Python script to sort lines of a file
When you manage files in Python programming project, and especially data, it is useful to sort the lines of a text file. It can be done by reading the file into a list, sorting the list, and then writing the list back to the file. It is important to note that this will sort the lines in alphabetical order by default. You can also use the sorted()
function with a key function to sort the lines in a different order.
with open("example.txt", "r") as file: lines = file.readlines() lines.sort() with open("example.txt", "w") as file: file.writelines(lines) print("Lines sorted successfully.")
5.7 Extract specific lines from a txt file
Extracting specific lines from a text file can be done by reading the file into a list, and then using list slicing to extract the specific lines. As you can see, in this example, the start
and end
variables represent the starting and ending line numbers to extract from the text file. The extracted lines are then written to a new file called new_file.txt.
with open("example.txt", "r") as file: lines = file.readlines() extracted_lines = lines[start:end] with open("new_file.txt", "w") as file: file.writelines(extracted_lines) print("Lines extracted successfully.")
This tutorial on files is very useful on programming projects using a large number of files, and it is using the Python strings to handle the directories, the files and content.
Conclusion on managing text files in Python
In conclusion, manage text files in Python is a simple and efficient process that can be done using the built-in functions and libraries such as open, read, write, and close. The use of the pandas library can also be useful for handling larger and more complex files.
By understanding the basic concepts and techniques for working with text files, you can easily develop your own programs and scripts to automate tasks and manipulate data. With this knowledge, you can now begin to explore the vast possibilities that text file management offers in the field of data science, machine learning, and data analysis.
Be the first to comment